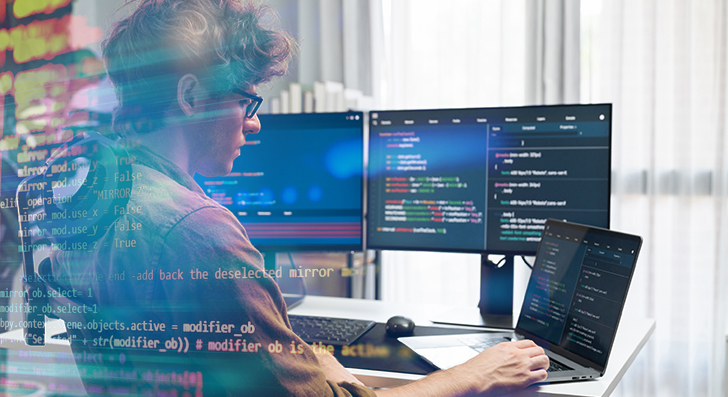
Scalability suggests your software can tackle expansion—far more consumers, more details, plus more website traffic—with no breaking. As being a developer, building with scalability in mind will save time and anxiety afterwards. Below’s a clear and simple guidebook that will help you get started by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component within your program from the start. Several programs are unsuccessful once they improve quickly for the reason that the initial structure can’t manage the additional load. Being a developer, you need to Consider early regarding how your program will behave stressed.
Start by planning your architecture to be versatile. Stay clear of monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Every single module or company can scale on its own without having impacting The complete system.
Also, take into consideration your databases from working day a single. Will it need to have to take care of one million customers or perhaps 100? Select the suitable type—relational or NoSQL—according to how your details will grow. Strategy for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t produce code that only operates underneath latest disorders. Think about what would happen In case your user base doubled tomorrow. Would your application crash? Would the databases slow down?
Use design patterns that support scaling, like information queues or party-pushed devices. These enable your application take care of far more requests without the need of having overloaded.
After you Establish with scalability in your mind, you're not just getting ready for success—you might be cutting down long run problems. A perfectly-prepared program is easier to take care of, adapt, and improve. It’s superior to organize early than to rebuild later.
Use the Right Databases
Deciding on the appropriate database is a vital Component of creating scalable applications. Not all databases are crafted precisely the same, and using the Improper you can sluggish you down or perhaps cause failures as your application grows.
Begin by being familiar with your knowledge. Is it really structured, like rows in the table? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are generally powerful with interactions, transactions, and consistency. In addition they help scaling procedures like browse replicas, indexing, and partitioning to deal with more targeted traffic and information.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, look at your read and generate patterns. Do you think you're doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Investigate databases that may take care of superior write throughput, and even celebration-centered information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Believe in advance. You might not require Superior scaling characteristics now, but deciding on a databases that supports them usually means you won’t require to switch later.
Use indexing to speed up queries. Stay clear of unnecessary joins. Normalize or denormalize your data based on your accessibility patterns. And often check database functionality while you increase.
In a nutshell, the ideal databases relies on your application’s composition, velocity desires, And just how you be expecting it to increase. Just take time to choose properly—it’ll help save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly created code or unoptimized queries can slow down general performance and overload your procedure. That’s why it’s essential to Make productive logic from the start.
Start by crafting clear, straightforward code. Steer clear of repeating logic and take away anything avoidable. Don’t select the most complicated solution if a straightforward one particular functions. Keep the features brief, concentrated, and simple to check. Use profiling applications to seek out bottlenecks—areas where your code can take also extensive to run or utilizes far too much memory.
Up coming, look at your databases queries. These usually gradual factors down more than the code by itself. Make certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches every thing, and as a substitute decide on specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, especially across significant tables.
Should you detect exactly the same knowledge remaining asked for again and again, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to exam with big datasets. Code and queries that perform wonderful with a hundred documents might crash once they have to manage one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These measures support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to handle more users and much more site visitors. If every little thing goes by means of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. Both of these equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out all of the function, the load balancer routes users to various servers according to availability. This suggests no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Equipment like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused promptly. When consumers request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database when. It is possible to serve it with the cache.
There's two frequent types of caching:
one. Server-facet caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces databases load, improves velocity, and tends to make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does modify.
Briefly, load balancing and caching are easy but highly effective equipment. Alongside one another, they help your application tackle a lot more people, stay quickly, and Get well from problems. If you plan to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need applications that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you may need them. You don’t really have to buy components or guess future capacity. When visitors will increase, it is possible to insert additional methods with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it needs to operate—code, libraries, options—into a single unit. This makes it easy to maneuver your app in between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app takes advantage of many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single element of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent parts of your application into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you could scale quickly, deploy effortlessly, and Get better swiftly when complications take place. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, cut down threat, and assist you remain centered on setting up, not fixing.
Watch Everything
When you don’t keep track of your application, you gained’t know when matters go Incorrect. Checking can help you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Applications like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your application far too. Regulate how much time it's going to take for users to load pages, how often errors occur, and exactly where they take website place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring within your code.
Put in place alerts for critical challenges. One example is, If the reaction time goes earlier mentioned a limit or even a support goes down, you ought to get notified right away. This assists you repair issues fast, normally right before people even observe.
Monitoring can also be useful after you make variations. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it leads to serious hurt.
As your app grows, traffic and facts boost. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate equipment set up, you keep in control.
Briefly, monitoring helps you maintain your app trusted and scalable. It’s not just about recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for major businesses. Even smaller apps will need a strong foundation. By designing meticulously, optimizing sensibly, and using the suitable tools, you may build apps that mature efficiently without breaking under pressure. Start out small, Feel major, and Develop sensible.